Integrating external applications when using Slack as a communication tool can be very useful. Slack notifications for specific actions speed up workflow processes and aid in efficiency. For example, receiving a notification whenever a deployment has been made or a git pull request that is ready to be reviewed.
In this article, there will be an explanation of integrating a Laravel application with Slack for a contact form.
Step 1
Creating a Slack App
The first step is to create a Slack app. To do this, you can go to api.slack.com which is a platform that enables users to extend by adding additional components and automating workspaces.
For the contact form created an app called Develo Contact Form and connected it to our personal workspace.
Activating incoming Webhooks for Slack app
Once the Slack app has been created it is essential to add Incoming Webhooks.
Incoming Webhooks are web services that can be called with a JSON payload. Their purpose is to accept data from other applications. Each Incoming Webhook must be able to generate a dynamic URL. For example, the Develo Contact form has a dynamic URL for an Incoming Webhook specific to the application.
Step 2.2
After adding the Incoming Webhook it can be tested in the application, in this instance the Laravel application. The Slack App provides a sample curl request to post to the channel that the Webhook is connected to.
This sample curl request can be pasted into the terminal inside of the Laravel application. If the Incoming Webhook connection is successful a “Hello World!” message should appear in the specific Slack channel.
Step 3.1
Configuring incoming webhook for Slack app in Laravel Application
The first step is to install Slack notification channel using composer by running the command:
composer require laravel/slack-notification-channel
When using the slack-notification-channel package it allows you to easily send notifications to a Slack channel using Laravel’s built-in notification system.
Once the Slack notification is installed the next step is to run the command:php artisan make:notification SendNotification
php artisan make:notification SendNotification
This will create a Notifications folder inside the app folder, the next step is to configure the SendNotification file with the code below:
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Messages\SlackMessage;
class SendNotification extends Notification
{
use Queueable;
private $contact;
public function __construct($contact)
{
$this->contact = $contact;
}
public function via($notifiable)
{
return ['slack'];
}
public function toSlack($notifiable)
{
return (new SlackMessage)->content($this->contact->name . "\n" .
$this->contact->email. "\n" . $this->contact->company. "\n" .
$this->contact->message);
}
}
One of the key aspects to note is that in the public function to Slack, the notification should return every element within the contact form in this case: name, email, company and message.
The key information in the contact form that users need to complete is referenced in the SendNotification.php file:
Step 3.2
To ensure that the right content is sent from the completed contact form using the Laravel application we must configure the Incoming Webhook using the right URL. To do this copy the Webhook URL in the Slack app for Incoming Webhooks.
The Webhook URL ensures that the Incoming Webhook is connected to the Laravel application.
For this Laravel Application we are connecting the contact form to Slack so that it can receive notifications whenever they are completed. We add the Webhook URL to app/Models/Contact.php inside of the public function routeNotificationForSlack.
Step 3.3
The final step is to configure app/HTTP/Controllers/ContactController.php file and sending notification from inside the controller. By importing the SendNotification class that we created previously.
Send notification from within the controller:
Summary
Understanding how to integrate Slack notifications using a Laravel application is useful in the following ways:
- Saves time by having notifications sent to Slack informing users of completed actions rather than having to check the database or emails.
- Creates efficient workflows without having to go outside of Slack to check for completed actions.
- Enables effective communication by not missing any important messages that have been sent either through a contact form or any other server-sidepost requests.
If you'd like to chat more about this with one of our Laravel developers, get in touch today!
Are you a Laravel Developer looking for more Laravel resources?
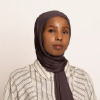
Zeinab Anshur
Quality Assurance Engineer
Zeinab has been a Quality Assurance Engineer working on eCommerce projects since 2023 and joined Develo the same year. Her favourite aspect of web development is creating an impact on clients’ businesses through meticulous test plans for successful launches. Her favourite aspect of Magento is creating comprehensive test plans to aid the running of seamless projects, and her favourite achievement from her time at Develo so far is testing the websites of household-named brands across industries. Outside of work, she enjoys travelling and hiking in country parks.
Develo is a leading Magento agency and eCommerce web development company based in Birmingham, in the UK, serving clients globally since 2010. We provide our Learn articles to share knowledge of magento web development, web app development and more to the wider web developer community. To extend your team with our white label web developers, collaborate or consult with us on your project contact us here.