Vuex! Our state management hero for Vue.
In this guide, we’re going to explain the concepts of state management in Vue, what is Vuex, the basics of it works.
After this, you’ll be ready to integrate Vuex into a new or existing Vue App!
As our requirements grow for our applications, they become increasingly complex, managing the state in an appropriate way is a big part of this complexity problem that can be quickly made easier using Vuex.
We’re going to assume you’re familiar with Vue already, if not, get yourself up to scratch with the basics here: https://vuejs.org/v2/guide
What is State Management?
State management is simply the way you handle your application state and data. There are certain patterns you can adhere to for better managing data and state across your application. Sometimes, this also is wrapped up in a library (like Vuex!) that will help you implement it.
In Vue, you’ll already be familiar with managing the application state at a basic level, you do this most the time by passing data to components via Props and emitting and catching Events across your App. This is everyday Vue stuff. However, for anything larger than an “advanced todo list” App (or a medium-to-large scale application in Vue), you’ll most likely come across some questionable decisions and repetition you’ve had to make in the way your handling the state of data shared across your App and advanced Components.
What is Vuex?
A great explanation taken from the official Vuex docs itself;
“Vuex is a state management pattern + library for Vue.js applications. It serves as a centralized store for all the components in an application, with rules ensuring that the state can only be mutated in a predictable fashion.”
What does it solve?
An example of how quickly things can spiral out of control is a shopping cart in Vue. In standard Vue, you’d probably end up manually emitting or passing data via props for our cart data changes to multiple components across our application (eg, header, product page, wishlist, cart page) Manually write some code to then catch these events if required and to update our local data for that component and let Vue render it again, displaying the updated cart contents/quantities/pricing etc. That’s a lot of important data to keep up to date across multiple places. Sometimes even passing data via Props isn’t an option, as some applications can be structured in all sorts of ways, so sometimes relying on mainly emitting events with your data as your single source of truth which isn’t great at all.
The more components you have, the more complex the interactions between these and the data it may share becomes inefficient and messy very quickly.
Vuex to the rescue 
Vuex helps by giving you an entire library (or system) you can use to easily keep the state/data of your application consistent and accessible easily through all of your components while ensuring updates to it are predictable through “mutations”
Using Vuex does complicate your application a little bit though – but in a good way! It’s a trade-off that you’ll have to deal with initially. Speed vs Complexity. It will slow you down at the start, but once you’ve got your head around its concepts and how it works, this slowdown is suddenly made up by all the help and stability it provides for your application. This helps prevent many of the issues of inconsistency in data and state that you can find in some larger Vue projects not using Vuex or a decent state management pattern. … So for a better understanding of Vuex, let’s dive in and explain the concepts of Vuex.
How does it work?
We can create a store in Vuex that gives us a place for us to put data, state and information that we want to be accessible across our application, an ideal use for this would be a place to store, a list of “todos”, and their done status for example.
But for demo purposes, let’s stick to an even simpler example, lets setup a basic Vue App and create a simple Vuex store that holds information for a number “count”.
You can install Vuex via the following methods:
NPM
npm install vuex --save
Yarn
yarn add vuexarn
CDN
https://unpkg.com/vuex
Once that’s done, you can import it and let Vue know we’re going to use it in this application:
Note: If you use Vue/Vuex via the CDN you can skip this import step.
For more in depth installation instructions see: https://vuex.vuejs.org/installation.html
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
Let’s create our basic Vue application and Vuex store.Want to learn more about our services or speak to us about a project?
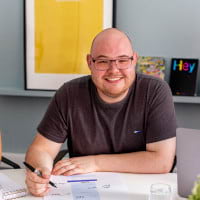
Mike Sheward
Technical Director
Mike is our Technical Director who has worked on eCommerce projects since 2014 and has been with Develo since 2015. He became certified in Adobe Commerce in 2023. Mike’s favourite aspect of web development is tackling intricate eCommerce challenges with the bespoke platform Magento/Hyvä, and he tells us his best achievement from working at Develo is fostering a culture of growth and innovation within our team. Outside of work, he can be found spending time with his family, baking and walking his dogs.
Develo is a leading Magento agency and eCommerce web development company based in Birmingham, in the UK, serving clients globally since 2010. We provide our Learn articles to share knowledge of magento web development, web app development and more to the wider web developer community. To extend your team with our white label web developers, collaborate or consult with us on your project contact us here.