What is a Trait
Traits are a handy way of reusing code in PHP, another way to help keep your code DRY (or “don’t repeat yourself”). If you’ve used whats commonly referred to as Mixins in some languages and frameworks, you’ll probably be familiar with Traits. It’s great that we can write some code, reuse that again and again throughout our codebase more efficiently, meanwhile – It’s good practice and helps us maintain the codebase easier, so yay for Laravel Traits / PHP Traits!
How using Traits help us in Laravel
In Laravel, you may of come across a variety of “out the box” traits that you can use, one of the most popular ones you may see across your Models is the Soft Deletes Trait. In short – using this trait allows you to “delete” your model, but without removing it entirely from the database. Utilizing a column in the database called “deleted_at” to “soft” delete the model when the trait is used. This can be useful if you frequently delete and restore models.
As you can see, using traits in your projects can be an easy way to create and add impressive reusable functionality to your project.
How to avoid abusing Traits
As traits allow you to easily add another set of functions to your classes, they may unfortunately become the place to be when you just want to add another little bit of shared functionality here and there if your not careful.
This is not a problem with Traits themselves, but more so how they are used. In your project, be careful to not let them get bloated with functionality that doesn’t concern them. Don’t add unnecessary code to a trait that doesn’t need to be there or can be abstracted away into another Class or Trait. Where possible, try and stick to the Single Responsibility Principle.
An example Image/File upload Laravel Trait
Why do we need this Trait
Majority of Laravel applications have a form somewhere with an image or file upload field in it, perhaps in an admin panel, or a form visible to the public, like changing your profile picture. So this becomes a great example for creating your first Trait.
Our new Trait would be responsible for getting the file upload from the request, checking if it’s valid, storing it and then returning the newly stored file path. It’s a small but handy trait to have – without using it, how many times would you have to rewrite the same code to handle this file upload functionality – in the create form? the edit form? and then for multiple occurances of a file upload in your forms? It can easily mount up.
Creating our Trait
Creating the Trait is easy! I chose to store all my traits in folder called Traits (usually located at App/Traits), using the namespace App\Traits, this way all my traits are easy to find. You can organise them in subfolders too.
So let’s go ahead and create the basic structure of our Trait. It will have a function called verifyAndStoreImage so that when our Trait is used, it’s clear what this function is expected to do.
namespace App\Traits;
trait StoreImageTrait {
public function verifyAndStoreImage() {
}
}
There’s not much code required to create a Trait, so it’s really easy to begin creating more Traits for your project where applicable.
We will fill out the rest of our trait, to include some basic file validation, storing the file locally, and then returning the file upload path.
namespace App\Traits;
use Illuminate\Http\Request;
trait StoreImageTrait {
/**
* Does very basic image validity checking and stores it. Redirects back if somethings wrong.
* @Notice: This is not an alternative to the model validation for this field.
*
* @param Request $request
* @return $this|false|string
*/
public function verifyAndStoreImage( Request $request, $fieldname = 'image', $directory = 'unknown' ) {
if( $request->hasFile( $fieldname ) ) {
if (!$request->file($fieldname)->isValid()) {
flash('Invalid Image!')->error()->important();
return redirect()->back()->withInput();
}
return $request->file($fieldname)->store('image/' . $directory, 'public');
}
return null;
}
}
To use your newly created Trait in your code, is really simple too. You’ll have to import the Trait, but after that you can use it like any other function, you can even pass arguments into them too. We will use the StoreImageTrait (that we created above) in our example Post Controller.
namespace App\Http\Controllers\Admin;
use App\Models\Admin\Post;
use Illuminate\Http\Request;
use App\Traits\StoreImageTrait;
class PostController extends Controller
{
use StoreImageTrait;
...
}
Once our image upload trait has been imported, we can then use it in one of our methods, in this case, we want to add it to our Store method in the Post Controller
namespace App\Http\Controllers\Admin;
use App\Models\Admin\Post;
use Illuminate\Http\Request;
use App\Traits\StoreImageTrait;
class PostController extends Controller
{
use StoreImageTrait;
/**
* Store a newly created Post in storage.
*
* @param CreatePostRequest $request
*
* @return Response
*/
public function store(CreatePostRequest $request)
{
$formInput = $request->all();
$formInput['image'] = $this->verifyAndStoreImage($request, 'image', 'post');
$post = Post::create($formInput);
flash('Post saved successfully.')->success();
return redirect(route('admin.posts.index'));
}
}
Laravel Traits, the conclusion
We’ve learnt what a Trait is, and how using them can offer you an invaluable solution not only help you stop repeating the same code over and over again, but improving your code by using them, making it easier to create cleaner, more readable and maintainable code.
To work with our Laravel developers, get in touch today.
Why not check out our other Laravel articles?
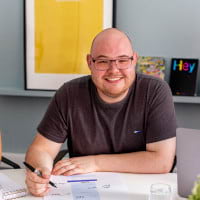
Mike Sheward
Technical Director
Mike is our Technical Director who has worked on eCommerce projects since 2014 and has been with Develo since 2015. He became certified in Adobe Commerce in 2023. Mike’s favourite aspect of web development is tackling intricate eCommerce challenges with the bespoke platform Magento/Hyvä, and he tells us his best achievement from working at Develo is fostering a culture of growth and innovation within our team. Outside of work, he can be found spending time with his family, baking and walking his dogs.
Develo is a leading Magento agency and eCommerce web development company based in Birmingham, in the UK, serving clients globally since 2010. We provide our Learn articles to share knowledge of magento web development, web app development and more to the wider web developer community. To extend your team with our white label web developers, collaborate or consult with us on your project contact us here.